Beego开发入门
安装
- 安装beego:
$ go get github.com/astaxie/beego
- 安装bee开发工具:
$ go get github.com/beego/bee
$ bee
Bee is a Fast and Flexible tool for managing your Beego Web Application.
USAGE
bee command [arguments]
AVAILABLE COMMANDS
version Prints the current Bee version
migrate Runs database migrations
api Creates a Beego API application
bale Transforms non-Go files to Go source files
fix Fixes your application by making it compatible with newer versions of Beego
dlv Start a debugging session using Delve
dockerize Generates a Dockerfile for your Beego application
generate Source code generator
hprose Creates an RPC application based on Hprose and Beego frameworks
new Creates a Beego application
pack Compresses a Beego application into a single file
rs Run customized scripts
run Run the application by starting a local development server
server serving static content over HTTP on port
Use bee help [command] for more information about a command.
ADDITIONAL HELP TOPICS
Use bee help [topic] for more information about that topic.
基本使用
创建项目
MVC类网页项目
创建普通的web服务类项目使用’bee new’命令,这类项目遵循通常的MVC代码架构。
$ bee new test/beego/bee/hello
______
| ___ \
| |_/ / ___ ___
| ___ \ / _ \ / _ \
| |_/ /| __/| __/
\____/ \___| \___| v1.9.1
2017/11/30 18:50:07 WARN ▶ 0001 You current workdir is not inside $GOPATH/src.
2017/11/30 18:50:07 INFO ▶ 0002 Creating application...
create Your_GOPATH/src/test/beego/bee/hello/
create Your_GOPATH/src/test/beego/bee/hello/conf/
create Your_GOPATH/src/test/beego/bee/hello/controllers/
create Your_GOPATH/src/test/beego/bee/hello/models/
create Your_GOPATH/src/test/beego/bee/hello/routers/
create Your_GOPATH/src/test/beego/bee/hello/tests/
create Your_GOPATH/src/test/beego/bee/hello/static/
create Your_GOPATH/src/test/beego/bee/hello/static/js/
create Your_GOPATH/src/test/beego/bee/hello/static/css/
create Your_GOPATH/src/test/beego/bee/hello/static/img/
create Your_GOPATH/src/test/beego/bee/hello/views/
create Your_GOPATH/src/test/beego/bee/hello/conf/app.conf
create Your_GOPATH/src/test/beego/bee/hello/controllers/default.go
create Your_GOPATH/src/test/beego/bee/hello/views/index.tpl
create Your_GOPATH/src/test/beego/bee/hello/routers/router.go
create Your_GOPATH/src/test/beego/bee/hello/tests/default_test.go
create Your_GOPATH/src/test/beego/bee/hello/main.go
2017/11/30 18:50:07 SUCCESS ▶ 0003 New application successfully created!
Web service类项目
如果项目是web service类型的则可以使用’bee api’来创建,这类项目创建完后比’bee new’类的项目省略’static’及’views’目录部分的内容。
运行项目
使用bee运行项目也非常简单,直接在项目代码目录下运行’bee run’即可,运行完后
$ bee run
______
| ___ \
| |_/ / ___ ___
| ___ \ / _ \ / _ \
| |_/ /| __/| __/
\____/ \___| \___| v1.9.1
2017/11/30 19:11:02 INFO ▶ 0001 Using 'hello' as 'appname'
2017/11/30 19:11:02 INFO ▶ 0002 Initializing watcher...
2017/11/30 19:11:03 SUCCESS ▶ 0003 Built Successfully!
2017/11/30 19:11:03 INFO ▶ 0004 Restarting 'hello'...
2017/11/30 19:11:03 SUCCESS ▶ 0005 './hello' is running...
2017/11/30 19:11:03 [I] [asm_amd64.s:2337] http server Running on http://:8080
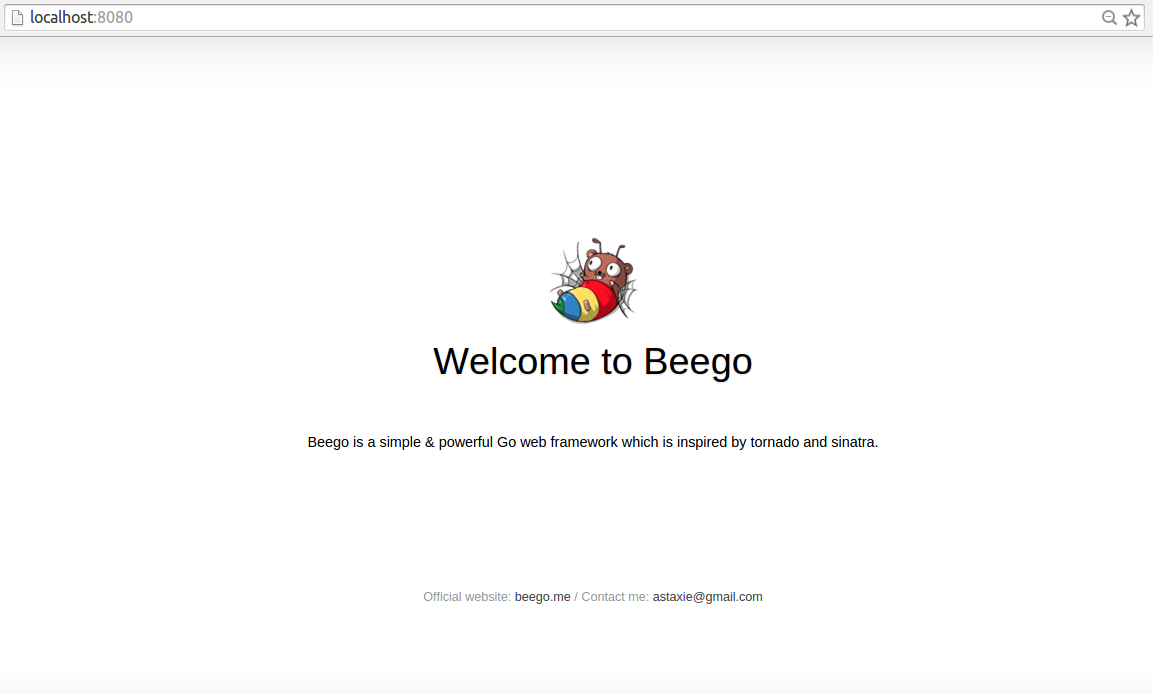
进阶开发
Route
项目创建后的router文件在“routers/router.go”
1package routers
2
3import (
4 "wangq/test/beego/bee/hello/controllers"
5 "github.com/astaxie/beego"
6)
7
8func init() {
9 beego.Router("/", &controllers.MainController{})
10}
创建一条router记录的方法是调用“beego.Router”方法,并提供两个参数:
- URI:请求的URI记录,比如”/”
- Controller:处理该请求的Controller
Controller
bee创建的项目controller位于“controllers/default.go”文件中。
1package controllers
2
3import (
4 "github.com/astaxie/beego"
5)
6
7type MainController struct {
8 beego.Controller
9}
10
11func (c *MainController) Get() {
12 c.Data["Website"] = "beego.me"
13 c.Data["Email"] = "astaxie@gmail.com"
14 c.TplName = "index.tpl"
15}
项目的’MainController’继承自’beego.Controller’,’beego.Controller’中定义了interface ‘ControllerInterface’用于处理HTTP请求,这些接口包括”Init, Prepare, Post, Get, Delete, Head”等,因此’MainController’可以通过实现这些方法来处理相应的请求。
在默认创建的controller中包括了’Get()‘方法,该方法用来处理HTTP ‘GET’请求。
Get()方法:
c.Data: 处理方法主要是利用’c.Data’这个map(数据类型为:map[interface{}]interface{})来存放返回的数据,其中的信息可以被template引用并render。
c.TplName: 指定template文件名字,如果不指定这个名字那么beego会尝试使用’controller/method_name.tpl’名字的文件作为模版。
View
beego使用go内建的html/template模版引擎,可以参考这个使用方法。
下面是例子模版,其中’{{.Website}}‘及’{{.Email}}‘是通过Controller的Data map提供给模版的参数。
...
<body>
<header>
<h1 class="logo">Welcome to Beego</h1>
<div class="description">
Beego is a simple & powerful Go web framework which is inspired by tornado and sinatra.
</div>
</header>
<footer>
<div class="author">
Official website:
<a href="http://{{.Website}}">{{.Website}}</a> /
Contact me:
<a class="email" href="mailto:{{.Email}}">{{.Email}}</a>
</div>
</footer>
<div class="backdrop"></div>
<script src="/static/js/reload.min.js"></script>
</body>
</html>
Model
GO语言相关的几个常用数据库支持如下:
- MySQL:github.com/go-sql-driver/mysql
- PostgreSQL:github.com/lib/pq
- Sqlite3:github.com/mattn/go-sqlite3
beego架构
工作流程: